はじめに
標準機能の頂点番号の文字サイズが小さいので、文字サイズを変えられるようにするアドオン
ついで、メニュー階層が深い機能の表面化
使い方
3Dビューポートで N キー。サイドメニューに「PgToys」が追加されます
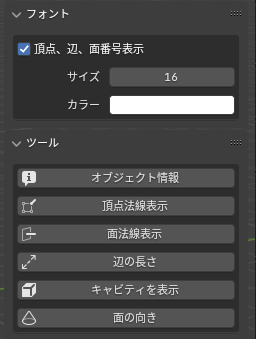
ダウンロード
更新情報
Ver 1.1
- パネルをフォントとツールに分割
- 辺の長さを追加
- エッジの強調(キャビティ)を追加
- 面の向きを追加
標準機能の頂点番号の文字サイズが小さいので、文字サイズを変えられるようにするアドオン
ついで、メニュー階層が深い機能の表面化
3Dビューポートで N キー。サイドメニューに「PgToys」が追加されます
Ver 1.1
コメント